If you’re an IT professional and haven’t dipped your toes into the world of PowerShell yet, it’s time to roll up your sleeves and get cracking. Why? Because PowerShell isn’t just a tool—it’s the tool. Think of it as your digital Swiss Army knife, capable of automating tasks, managing systems, and making you look like an IT wizard to your colleagues.
A Warning!
Never, Ever execute Code you do not trust and know what it does! With PowerShell, you can do anything you wish… especially with Administrative Permissions. Therefore, always look at what it does before blindly executing it.
Since most of my Solutions will be via PowerShell, here you have a simple introduction:
What Even Is PowerShell?
Let’s start with the basics. PowerShell is a task automation and configuration management framework from Microsoft. Translation? It’s like Command Prompt, but with a cape and superpowers. Built on the .NET framework, it’s designed to let you automate just about anything in the Windows ecosystem—and beyond. Oh, and it’s open-source, which means you can also flex your cross-platform muscles on macOS and Linux. You’re welcome.
Syntax: The Verb-Noun Rule
PowerShell commands, known as cmdlets (pronounced “command-lets”), follow a simple and consistent naming convention: Verb-Noun. This makes it easy to understand what a cmdlet does at a glance. Here are some common examples:
Get-Process
: Fetches information about running processes.Stop-Service
: stops a running Service.Set-ExecutionPolicy
: Configures script execution policies.New-Item
: Creates new files, directories, or other items.
If PowerShell had a mantra, it’d be: Get it, Set it, New it. The first part (the verb) tells you what action will happen, and the second part (the noun) tells you what’s being acted upon. Simple, right?
Variables: Your Data Sidekick
Before diving deeper into PowerShell, let’s talk about variables. Think of variables as labeled boxes where you can store information—numbers, text, objects, or even the output of cmdlets. In PowerShell, variables always start with a dollar sign ($
).
Here’s an example:
# Assigning values to variables
$Name = "PowerShell"
$Age = 19
# Using variables
Write-Host "Welcome to $Name, a tool that’s $Age years old!"
This will output:
Welcome to PowerShell, a tool that’s 19 years old!
PowerShell variables are dynamic, meaning their type (string, integer, object, etc.) is determined based on the assigned value. You can even assign cmdlet outputs to variables:
# Storing the output of a cmdlet
$Services = Get-Service
# Displaying the first service in the list
Write-Host $Services[0]
Pro Tip: Use descriptive variable names to make your scripts easier to read and maintain.
Getting Help: Your Built-In Lifeline
PowerShell comes with a fantastic built-in help system. Whenever you’re unsure what a cmdlet does or how to use it, the Get-Help
cmdlet is your best friend. For example:
Get-Help Get-Process
This will display a detailed description of the Get-Process
cmdlet, including its syntax, parameters, and examples. Want even more detail? Add the -Detailed
or -Examples
switch:
Get-Help Get-Process -Detailed
Get-Help Get-Process -Examples
Pro Tip: If the help files seem outdated, run this to update them:
Update-Help
Script Files: Your Automation Toolkit
PowerShell scripts are where the magic truly happens. They are simply text files with the .ps1
extension, containing one or more cmdlets, variables, and logic to perform tasks automatically. Let’s explore how to create and execute script files.
Creating a Script File
- Open your favorite text editor (e.g., Notepad, VS Code, or the PowerShell ISE).
- Write your PowerShell code. For example:
# A script to greet the user and list running processes
$UserName = Read-Host "Enter your name"
Write-Host "Hello, $UserName! Here are the processes running on your machine:"
Get-Process
- Save the file with a
.ps1
extension, such asGreetUser.ps1
.
Running a Script File
To execute your script, open PowerShell, navigate to the directory where the script is saved (cd stands for “Change Directory”):
cd C:\User\kMarflow\Documents\tutorials\Powershell
and run it:
.\GreetUser.ps1
Execution Policy
If you encounter an error like script execution is disabled
, it’s due to the script execution policy. To allow script execution, run:
Set-ExecutionPolicy -Scope CurrentUser -ExecutionPolicy RemoteSigned
Choose an appropriate execution policy based on your security needs. The RemoteSigned
Policy allows locally created scripts to run while requiring a digital signature for scripts downloaded from the internet.
Pro Tip: Always keep your scripts organized and well-commented to make them easier to maintain and debug.
Temporarily bypass Execution Policy
Alternatively, you can also just bypass the execution policy for the current PowerShell Session:
Set-ExecutionPolicy -ExecutionPolicy Bypass
Your First PowerShell Script
Let’s flex those newfound skills with a simple script. PowerShell scripts are just text files with a .ps1
extension. Open your favourite text editor (or the PowerShell ISE) and type this:
# A simple script to list all services on your machine
Get-Service | Out-File -FilePath C:\temp\ServiceList.txt
Write-Host "Service list saved to C:\temp\ServiceList.txt!"
Save it as ListServices.ps1
, then run it in PowerShell by navigating to its directory and typing:
cd C:\User\kMarflow\Documents\tutorials
.\ListServices.ps1
Boom! You just created and executed your first script. How’s that for a productivity boost?
Understanding Error Messages: Don’t Panic
PowerShell’s error messages might seem intimidating at first, but they’re actually quite helpful if you know how to read them. Let’s look at an example:
Get-Process -Name NonExistentProcess
This will throw an error like:
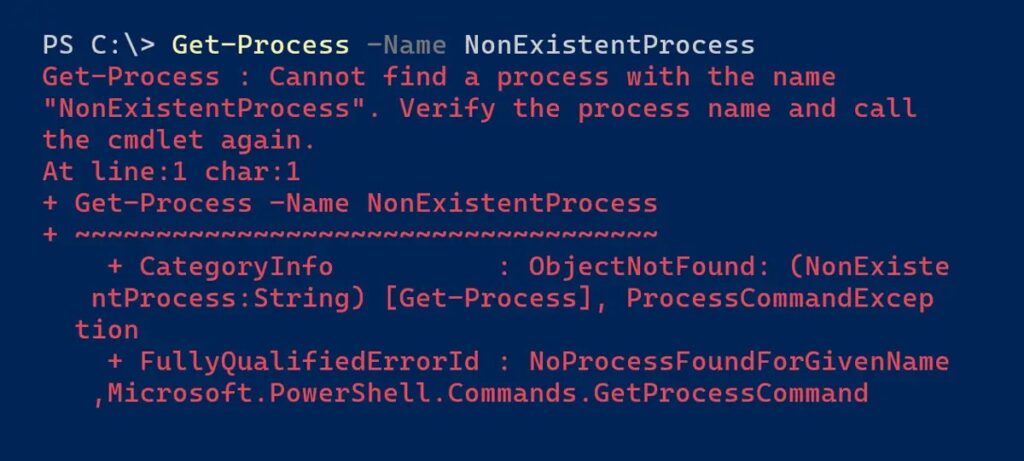
Key parts of the error:
- Cmdlet Name: Shows which cmdlet caused the issue (
Get-Process
). - Description: Explains what went wrong (Cannot find a process with the name).
- Actionable Advice: Often suggests what to fix (verify the process name).
Pro Tip: You can use the $Error
variable to see a list of recent errors and dig into their details. This Variable is a List, where calling $Error
will return the whole object. To get a certain position of that list, you can use the index $Error[0]
(In programming, we start to count from 0, so the first/latest element here will be in position 0). For example:
$Error[0]
This gives you a detailed breakdown of the last error, helping you troubleshoot like a pro.
Tips for the PowerShell Newbie
Tab Completion: Don’t know the full command? Start typing and hit Tab
. PowerShell will finish your sentence like it’s your best friend.
Learn by Doing: Start small. Automate something you hate doing manually. (Looking at you, monthly file cleanup.)
Error Messages Are Your Friend: PowerShell’s error messages are usually clear and helpful. Use them to learn and improve.
Closing Thoughts
PowerShell isn’t just a tool—it’s a mindset. It’s about taking control of your systems, streamlining your workflows, and impressing everyone with your automation chops. Sure, there’s a learning curve, but once you’re on the other side, you’ll wonder how you ever lived without it.
So, grab your favourite beverage (Coffee!… bucket-wise!), fire up PowerShell, and start exploring. Who knows? You might just fall in love… or at least get really good at saving time.
Start your PowerShell journey today and transform how you manage your systems.
Tip
To get more comfortable, just open a PowerShell session and try a few things like one of the following
cd $env:userprofile\Documents
dir
dir $env:windir
tree
whoami
New-Item -Type Directory -Path C:\temp
Get-CimInstance Win32_OperatingSystem
Get-CimInstance Win32_OperatingSystem | Format-Table -Wrap
Get-CimInstance Win32_OperatingSystem | Select-Object Caption, Version, SerialNumber
Get-CimInstance Win32_OperatingSystem | Select-Object Caption, Version, SerialNumber > "C:\temp\WinInfo.ext"
cat "C:\temp\WinInfo.ext"
Leave a Reply